Step 1: Make it
What is it?
With two micro:bits you can keep track of a precious belonging or pet using radio messages.
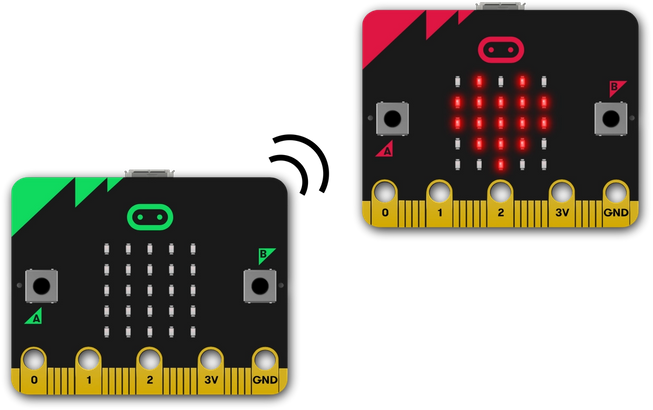
How it works
- This uses two programs, a transmitter (beacon) program and a receiver.
- Flash the transmitter program onto the first micro:bit, connect it to a battery pack and put it in or on your precious thing.
- Using an infinite loop, it broadcasts a low power ‘hello’ radio message on group 73 every 2 seconds. (You can use any radio group number you like between 0 and 255, just make sure that the receiver program uses the same number. Groups are like channels on TV or a walkie-talkie.)
- The receiver program will show you a heart on the LED display output for 1 second every time it receives a message on the same channel. Because we’re using a low-power transmitter you have to be quite close to the transmitter to see it, so you’ll know your precious thing is nearby.
What you need
- 2 micro:bits
- MakeCode or Python editor
- At least 1 battery pack
- something precious to keep track of
Step 2: Code it
Transmitter
Receiver
Step 3: Improve it
- Increase the range by increasing the power of the radio transmitter. The power can be any number from 0 to 7.
- Make a heart ‘beat’ on the transmitter’s LED display as well on the receiver.
- You could use these programs as a simple treasure hunt game – hide the transmitters and challenge a friend to find them.
This content is published under a Creative Commons Attribution-ShareAlike 4.0 International (CC BY-SA 4.0) licence.