Step 1: Make it
What is it?
Use several micro:bits to make a physical treasure hunt game using radio communication.
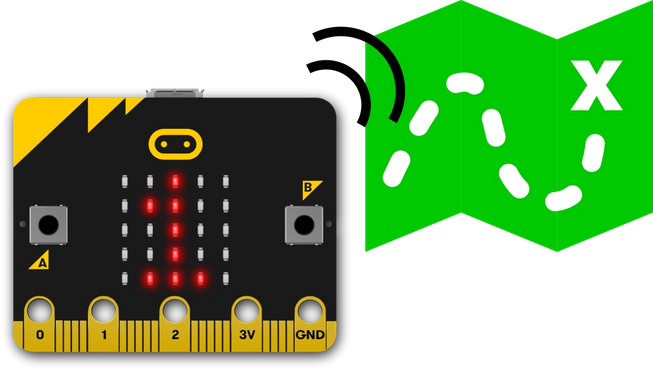
How it works
- Like the Heartbeat beacon project, this uses two different programs, one to transmit radio signals, and another to receive the signals.
- Flash the beacon (transmitter) program onto different micro:bits, making sure you change the number in the id variable so each one is different. The beacons briefly show their ID number on the display, so you know which is which. Attach battery packs and then hide the beacons which transmit their ID number every 200 milliseconds (0.2 seconds).
- Flash the receiver code on to micro:bits for the treasure hunters. This program can be the same for all receivers. When you approach a beacon, the receiver program shows the ID number it’s broadcasting. The display will blink when you are further away and become steady as you get closer.
- Make your own rules for the game – note the numbers down and where you found them, or collect the physical micro:bit beacons. Team with the most wins.
What you need
- Several micro:bits and battery packs
- MakeCode or Python editor
- a reasonably large space to hide beacons in – it could be indoors or outdoors
Step 2: Code it
Beacon / transmitter
Receiver
Step 3: Improve it
- Change the transmitter power to cover a larger or smaller area. The power can be any number from 0 to 7.
- Further reduce the radio power of the beacons making them hard to find – be careful not to place the micro:bits directly in any metal containers, but if you put one in a cardboard or plastic box and then partially cover the outside of the box in tin foil, for example, the radio signals will not travel as far.
- Transmitting radio signals uses more power, so you could make the beacon batteries last longer by increasing the delay between transmissions to more than 200 milliseconds.
This content is published under a Creative Commons Attribution-ShareAlike 4.0 International (CC BY-SA 4.0) licence.