Step 1: Make it
What is it?
Code your own electronic metronome that makes regular beats which you can slow down and speed up as you practice music.
Introduction
Coding guide
What you'll learn
- How to adjust the tempo of musical notes played by the micro:bit
- How to use input buttons and audio outputs to make a useful electronic device
How it works
- At the start of the program, it sets the tempo to 100 bpm - beats per minute. This is a standard way of measuring the tempo, or speed, of music.
- A forever loop keeps the micro:bit playing a short note and then resting for one beat.
- You can hear the sound by attaching headphones to pin 0 and GND or on the built-in speaker of the new micro:bit.
- Press button A to slow down the tempo by 5 bpm.
- Press button B to speed it up by 5 bpm.
- Pressing button A and B together shows the current tempo on the LED display output.
What you need
- A micro:bit
- MakeCode or Python editor
- battery pack (optional)
Step 2: Code it
Step 3: Improve it
- You may notice that it plays its rhythm slightly slower than an electronic instrument with the same BPM setting - this is because each loop takes one and one sixteenth of a beat, instead of just one beat.
- You could try and make it more accurate by adding up fractions of a beat to make one whole beat: 1/2 + 1/4 + 1/8 + 1/16 makes 15/16 to add on the 1/16th of a beat that you’re playing the tone for.
- 'Forever' blocks also add a bit of a delay, so using a ‘while true’ loop inside it will speed up your code and make it more accurate.
- The code video at the top of this page shows you how to do this.
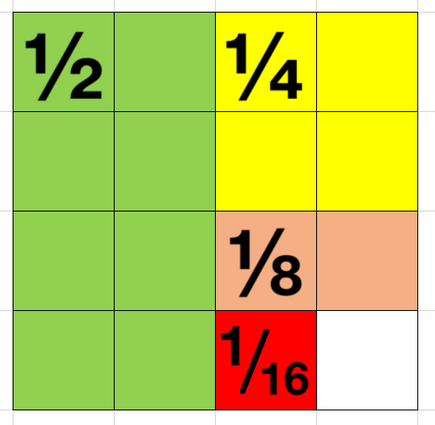
This content is published under a Creative Commons Attribution-ShareAlike 4.0 International (CC BY-SA 4.0) licence.