Step 1: Make it
What is it?
Make an electronic candle that you can blow out! The new micro:bit's built-in microphone detects the sound of your breath and turns the candle off - and on again.
Introduction
Coding guide
What you'll learn
- How to use random numbers to light random LEDs
- How to switch outputs in response to sensor inputs
- How to use Boolean logic to make a switch that toggles on and off when triggered by the same event
How it works
- A random number between 1 and 3 is stored in variable called flicker.
- This number is used to turn LEDs on and off at random to look like a flame flickering.
- The micro:bit's LEDs are arranged in a grid with columns and rows numbered from 0 to 4. The program plots (turns on) and un-plots (turns off) different LEDs in the top row depending on the random number stored in the flicker variable.
- Co-ordinates for the LEDs are always given with the column across first (the x-axis) and then the row up and down (the y-axis). The middle of the flame is at co-ordinate 2, 0.
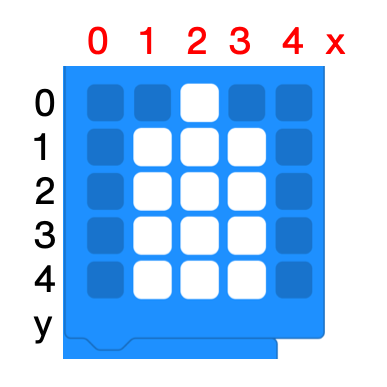
- A forever loop keeps the animation running.
- The program also uses a variable called lit to keep track of the whether the candle is lit or not. This is a Boolean variable. Boolean variables can only have two values: true (on) or false (off).
- When the microphone sensor detects a loud sound, for example when you blow on it, the code toggles the value of lit by setting it to be not lit. So, when you blow on the microphone, if lit is true, it becomes false and the screen is cleared, turning off the LEDs.
- If lit was false, it becomes true and we switch the animation back on.
What you need
- A micro:bit
- MakeCode or Python editor
- battery pack (optional)
Step 2: Code it
1from microbit import *
2import random
3
4lit = True
5
6while True:
7 if microphone.was_event(SoundEvent.LOUD):
8 lit = not lit
9 sleep(500)
10 if lit:
11 display.show(Image(
12 "00900:"
13 "09990:"
14 "09990:"
15 "09990:"
16 "09990"))
17 sleep(150)
18 flicker = random.randint(1, 3)
19 if flicker != 2:
20 display.set_pixel(2,0,0)
21 display.set_pixel(flicker,0,9)
22 sleep(150)
23 else:
24 display.clear()
Step 3: Improve it
- Create your own design for an animation to turn on or off using sound.
- You can make the 'on loud sound' block more or less sensitive by adding a 'set loud sound threshold' block to an ‘on start’ block. Find this under Input and '...more.' Use smaller numbers for quieter sounds, larger numbers for louder sounds.
- In Python, to change the threshold for loud sounds use
microphone.set_threshold(SoundEvent.LOUD, 128)
just before thewhile True:
loop, changing the number 128 to the sound value you want. This can be any number between 0 and 255, with 255 being the loudest sound.
This content is published under a Creative Commons Attribution-ShareAlike 4.0 International (CC BY-SA 4.0) licence.