Step 1: Make it
What is it?
A dice project that looks like a real die with patterns of dots instead of numbers.
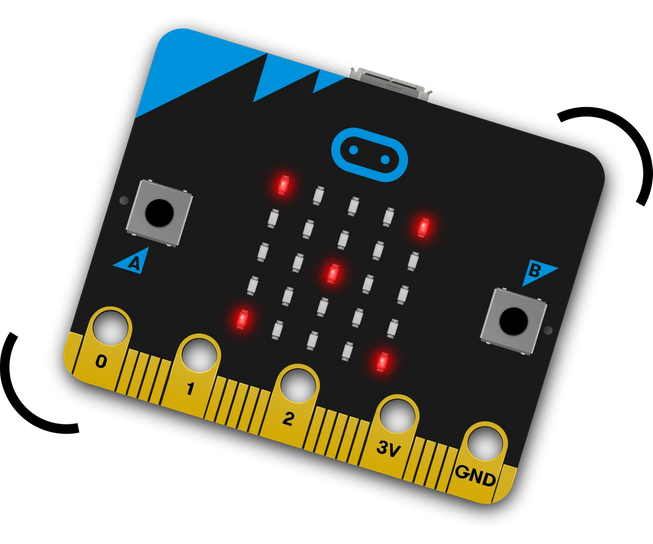
How it works
- Like the Dice project this uses the accelerometer input to trigger the creation of a random number between 1 and 6 and show it on the LED display output when you shake the micro:bit.
- Instead of showing a number, this program uses selection to show dots on the display to represent the numbers, looking like the dots on each face of real dice, depending on which random number was generated.
What you need
- micro:bit (or MakeCode simulator)
- MakeCode or Python editor
- battery pack (optional)
- squared paper for designing your own dice faces (optional)
Step 2: Code it
1from microbit import *
2import random
3
4while True:
5 if accelerometer.was_gesture('shake'):
6 number = random.randint(1, 6)
7 if number == 1:
8 display.show(Image(
9 "00000:"
10 "00000:"
11 "00900:"
12 "00000:"
13 "00000"))
14 elif number == 2:
15 display.show(Image(
16 "00000:"
17 "00000:"
18 "90009:"
19 "00000:"
20 "00000"))
21 elif number == 3:
22 display.show(Image(
23 "00009:"
24 "00000:"
25 "00900:"
26 "00000:"
27 "90000"))
28 elif number == 4:
29 display.show(Image(
30 "90009:"
31 "00000:"
32 "00000:"
33 "00000:"
34 "90009"))
35 elif number == 5:
36 display.show(Image(
37 "90009:"
38 "00000:"
39 "00900:"
40 "00000:"
41 "90009"))
42 else:
43 display.show(Image(
44 "90009:"
45 "00000:"
46 "90009:"
47 "00000:"
48 "90009"))
Step 3: Improve it
- Make the display clear after a few seconds to make the batteries last longer and to make it clear when you have rolled two numbers the same.
- Draw your own dot patterns to represent each number.
- Make it roll higher numbers. How would you represent them on the 5x5 LED grid display output?
This content is published under a Creative Commons Attribution-ShareAlike 4.0 International (CC BY-SA 4.0) licence.